While working on an e-commerce project last year, I came across this problem where a customer is presented with a quantity input field, which can only set the quantity in fixed multiples depending on few constraints.
I don’t remember the specifics of the constraints as I was reassigned to a different project, so I had to leave this problem midway without ever raising a pull request for it. Just thought I would share the solution I came up for it.
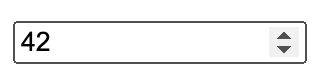
This is the input field the customer had to use, using the up/down arrow keys to set the quantities.
The constraint
The quantity should be a multiple of 4, 5, or 7.
What it means:
If the quantity is increased from 15 by clicking the up arrow once, then the next value set should be 16, as it is divisible by 4. Clicking it once more should set it to 20 as it is divisible by 5.
Solution
function getQuantityUpdaterFn( inputArray = [] ) {
let min = Math.min( ...inputArray );
return function( action ) {
return function* () {
while ( true ) {
if ( action === 'DEC' ) {
if ( min !== 0 ) {
min--;
}
} else if ( min === 0 ) {
min++;
}
if ( inputArray.filter( x => min % x === 0 ).length > 0 ) {
yield min;
}
if ( action === 'INC' ) {
min++;
}
}
}
}
}
let getQuantityUpdaterByAction = getQuantityUpdaterFn( [ 4, 5, 7 ] );
const increaseQuantity = getQuantityUpdaterByAction( 'INC' )();
const decreaseQuantity = getQuantityUpdaterByAction( 'DEC' )();
console.log( increaseQuantity.next().value ); // 4
console.log( increaseQuantity.next().value ); // 5
console.log( increaseQuantity.next().value ); // 7
console.log( increaseQuantity.next().value ); // 8
console.log( increaseQuantity.next().value ); // 10
console.log( increaseQuantity.next().value ); // 12
console.log( increaseQuantity.next().value ); // 14
console.log( increaseQuantity.next().value ); // 15
console.log( increaseQuantity.next().value ); // 16
console.log( increaseQuantity.next().value ); // 20
console.log( increaseQuantity.next().value ); // 21
console.log( decreaseQuantity.next().value ); // 20
console.log( decreaseQuantity.next().value ); // 16
console.log( decreaseQuantity.next().value ); // 15
console.log( decreaseQuantity.next().value ); // 14
console.log( decreaseQuantity.next().value ); // 12
console.log( decreaseQuantity.next().value ); // 10
console.log( decreaseQuantity.next().value ); // 8
Code language: JavaScript (javascript)
Now, this does not mean the solution above is the best out there. This was just an attempt using generators to solve this problem at hand.
What does the solution not do?
The solution works fine as long as we use the up/down arrows. However, it does not handle the situation when the quantity is directly typed in the input field.
Someday I will revisit and improve, until then see ya!
Leave a Reply