Alternatively, the title could be – How to disable the code editor in Gutenberg?
In WordPress, users can edit a specific block’s HTML by selecting Edit as HTML in the block’s More options Toolbar menu. (image below)
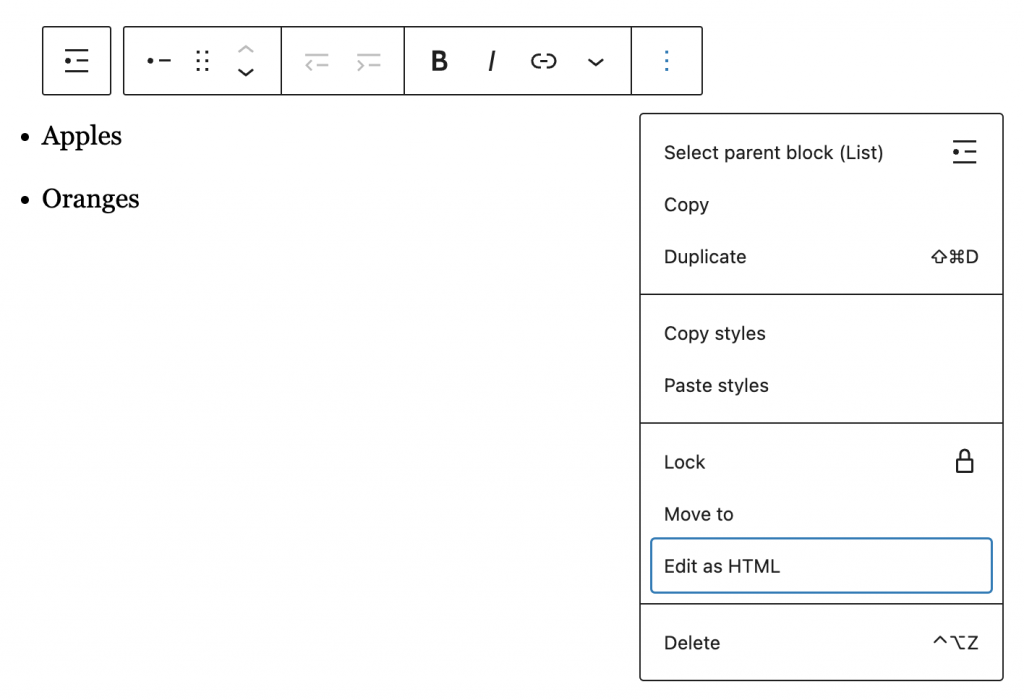
WordPress also provides a way to edit the entire post content as HTML by allowing users to switch from the Visual Editor to the Code editor. (image below)
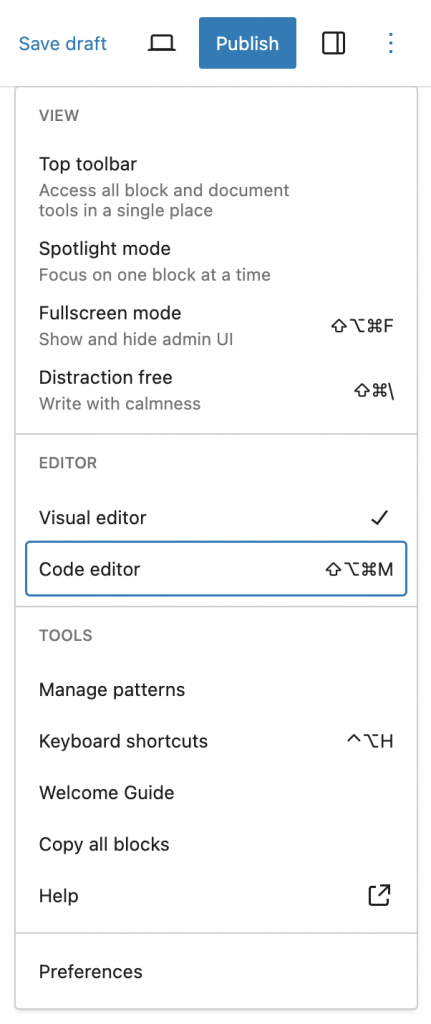
For a requirement where you don’t want to allow a user to edit the HTML, you can use either PHP or JavaScript to disable it.
PHP
The most straight forward way to do it is by using the block_editor_settings_all filter hook.
<?php
function my_plugin_disable_rich_editing( $editor_settings, $editor_context ) {
$editor_settings['codeEditingEnabled'] = false;
return $editor_settings;
}
add_filter( 'block_editor_settings_all', 'my_plugin_disable_rich_editing', 10, 2 );
Code language: PHP (php)
This is the simplest implementation which will disable code editing throughout the site.
In the example above, we are not using $editor_context
at all. This variable contains the WP_Post
object, which will be useful if you want to disable code editing conditionally, for example for a specific post type.
JavaScript
Since we don’t want to render anything, register a plugin:
import { registerPlugin } from '@wordpress/plugins';
const DisableCodeEditor = () => {
return null;
};
registerPlugin(
'disable-code-editor',
{
render: DisableCodeEditor
}
);
Code language: JavaScript (javascript)
Check if code editing is enabled for the editor:
import { registerPlugin } from '@wordpress/plugins';
import { useSelect, useDispatch } from '@wordpress/data';
const DisableCodeEditor = () => {
const isCodeEditingEnabled = useSelect(
__select => __select( 'core/editor' ).getEditorSettings().codeEditingEnabled
);
return null;
};
registerPlugin(
'disable-code-editor',
{
render: DisableCodeEditor
}
);
Code language: JavaScript (javascript)
Disable code editing (complete code):
import { useSelect, useDispatch } from '@wordpress/data';
import { useEffect } from '@wordpress/element';
const DisableCodeEditor = () => {
const isCodeEditingEnabled = useSelect(
__select => __select( 'core/editor' ).getEditorSettings().codeEditingEnabled
);
const { updateEditorSettings } = useDispatch( 'core/editor' );
useEffect( () => {
// Disable code editing if it is enabled.
if ( isCodeEditingEnabled ) {
updateEditorSettings( { codeEditingEnabled: false } );
}
}, [ isCodeEditingEnabled ] );
return null;
};
registerPlugin(
'disable-code-editor',
{
render: DisableCodeEditor
}
);
Code language: JavaScript (javascript)
In the images below, you can see the Edit as HTML and Editor options are no longer visible.
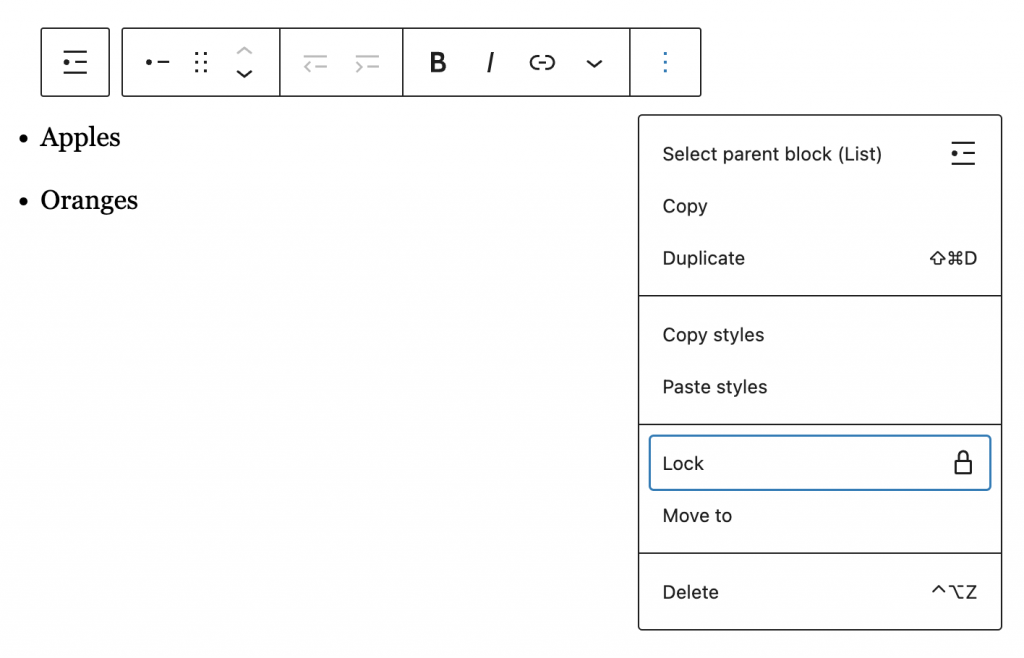
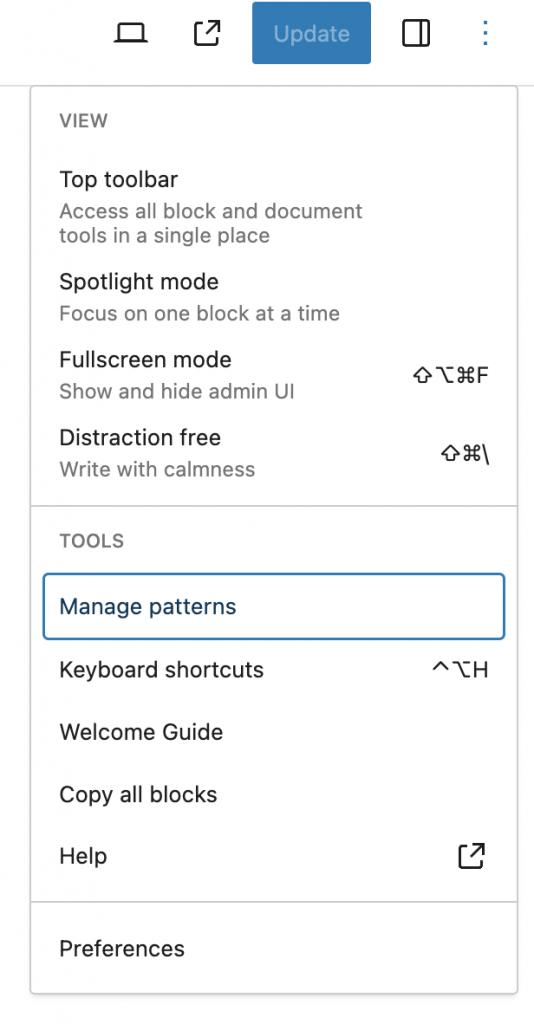
That’s it! Thanks for reading!
Leave a Reply