WordPress supports a wide list of sites that it can embed. This article will demonstrate how you can use Gutenberg’s built-in capability to display embed previews from of any of the supported sites. For the sake of learning, let’s set the following goal:
Goal: Create a block that has a URL input field. When a URL from any of the supported sites is entered, the block should display an embed preview.
Note: Our main focus is to show the embed preview in the Gutenberg editor, so we will cover just that.
Set up the skeleton of the component
import { useBlockProps } from '@wordpress/block-editor';
import { useState } from "@wordpress/element";
import { __ } from "@wordpress/i18n";
export default function Edit() {
/**
* @param url The URL which will be used for the embed.
* @param setUrl The function which will set the URL.
*/
const [ url, setUrl ] = useState( '' );
return (
<div { ...useBlockProps() }>
<div>
<label>
{ __( 'Input the URL:' ) }
<input type="url" onChange={ e => setUrl( e.target.value ) } />
</label>
</div>
<div>
{ __( 'Embed Preview:' ) }
</div>
</div>
);
}
Code language: JavaScript (javascript)
I have added some CSS to give it some basic structure and styling. This is how our skeleton Component looks like.
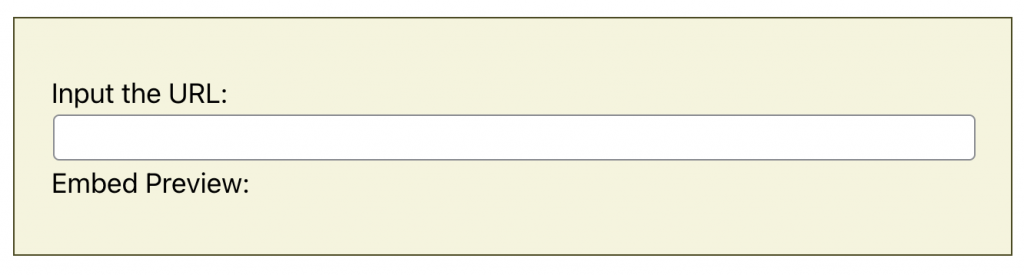
Fetch the embed preview
import { useBlockProps } from '@wordpress/block-editor';
import { useState } from "@wordpress/element";
import { __ } from "@wordpress/i18n";
import { useSelect } from "@wordpress/data";
export default function Edit() {
/**
* @param url The URL which will be used for the embed.
* @param setUrl The function which will set the URL.
*/
const [ url, setUrl ] = useState( '' );
const { preview } = useSelect( select => {
const { getEmbedPreview } = select( 'core' );
return {
preview: url.length ? getEmbedPreview( url ) : false,
}
}, [ url ] );
return (
<div { ...useBlockProps() }>
<div>
<label>
{ __( 'Input the URL:' ) }
<input type="url" onChange={ e => setUrl( e.target.value ) } />
</label>
</div>
<div>
{ __( 'Embed Preview:' ) }
{ preview && (
<div dangerouslySetInnerHTML={ { __html: preview.html } } />
) }
</div>
</div>
)
}
Code language: JavaScript (javascript)
Final result
Can the performance be optimized?
Yes. You can debounce the URL input field so that useSelect
doesn’t run on every character updated in the field.