Let’s create something simple and meaningless just for the sake of learning.
Goal: Create a Gutenberg plugin which registers a global shortcut – Ctrl + R
that changes the color of all text on the page to red.
Create a React Component that returns null
const MakeTextRedshortcut = () => {
return null;
}
Code language: JavaScript (javascript)
This component will later hold the logic for registering the shortcut, as well as using it. It returns null
because we don’t want anything to render on the page.
Register a Gutenberg plugin
import { registerPlugin } from '@wordpress/plugins';
const MakeTextRedshortcut = () => {
return null;
}
registerPlugin(
'global-text-color-change-plugin',
{
render: MakeTextRedshortcut
}
);
Code language: JavaScript (javascript)
We’ve named our plugin global-text-color-change-plugin
, you can name it whatever you want as long as the name doesn’t clash with an already registered plugin.
The plugin will render the MakeTextRedshortcut
component.
Register the shortcut
import { registerPlugin } from '@wordpress/plugins';
import { useDispatch } from "@wordpress/data";
const MakeTextRedshortcut = () => {
const { registerShortcut } = useDispatch( 'core/keyboard-shortcuts' );
registerShortcut( {
name: 'change-global-text-color-to-red-shortcut',
category: 'global',
description: 'Turns the color of all text on the screen to red',
keyCombination: {
modifier: 'ctrl',
character: 'r',
},
} );
return null;
}
registerPlugin(
'global-text-color-change-plugin',
{
render: MakeTextRedshortcut
}
);
Code language: JavaScript (javascript)
A shortcut is registered using the registerShortcut
method which takes an object with the following fields:
name
: This is the unique name given to the shortcut. You can name it anything you want as long as a shortcut isn’t already registered with the same name.category
: When we open Keyboard Shortcuts, our shortcut will be added under the Global shortcuts section. (See the image below)description
: A text to describe what the shortcut does.keyCombination
: Defines keys to be used for the shortcut sequence.
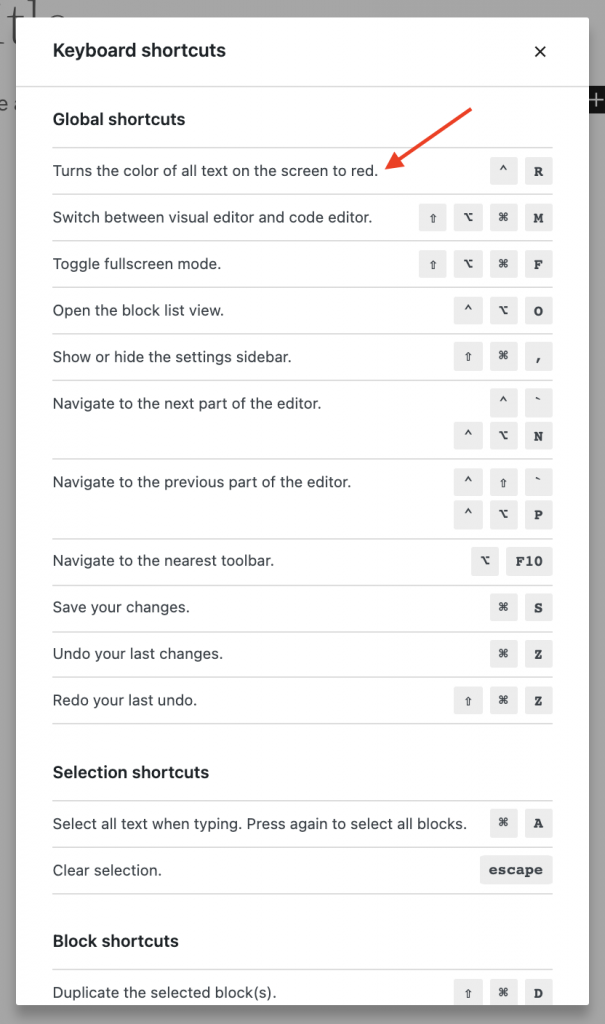
Define the functionality of the shortcut
We have registered the shortcut Ctrl + R
but we haven’t yet told Gutenberg what it does. To do this, we use Gutenberg’s React hook useShortcut()
import { registerPlugin } from '@wordpress/plugins';
import { useDispatch } from "@wordpress/data";
import { useShortcut } from '@wordpress/keyboard-shortcuts';
const MakeTextRedshortcut = () => {
const { registerShortcut } = useDispatch( 'core/keyboard-shortcuts' );
registerShortcut( {
name: 'change-global-text-color-to-red-shortcut',
category: 'global',
description: 'Turns the color of all text on the screen to red',
keyCombination: {
modifier: 'ctrl',
character: 'r',
},
} );
useShortcut(
'change-global-text-color-to-red-shortcut', // name of our shortcut.
() => {
// This function will run when Ctrl + R will be pressed.
jQuery( '*' ).css( 'color', 'red' );
}
);
return null;
}
registerPlugin(
'global-text-color-change-plugin',
{
render: MakeTextRedshortcut
}
);
Code language: JavaScript (javascript)
Can we optimize the performance a little bit?
Yes. We can memoize the callback for useShortcode since the functionality is same throughout the lifecycle.
import { registerPlugin } from '@wordpress/plugins';
import { useDispatch } from "@wordpress/data";
import { useShortcut } from '@wordpress/keyboard-shortcuts';
import { useCallback } from '@wordpress/element';
const MakeTextRedshortcut = () => {
const { registerShortcut } = useDispatch( 'core/keyboard-shortcuts' );
registerShortcut( {
name: 'change-global-text-color-to-red-shortcut',
category: 'global',
description: 'Turns the color of all text on the screen to red',
keyCombination: {
modifier: 'ctrl',
character: 'r',
},
} );
useShortcut(
'change-global-text-color-to-red-shortcut', // name of our shortcut.
useCallback( () => {
// This function will run when Ctrl + R will be pressed.
jQuery( '*' ).css( 'color', 'red' );
}, [] )
);
return null;
}
registerPlugin(
'global-text-color-change-plugin',
{
render: MakeTextRedshortcut
}
);
Code language: JavaScript (javascript)