Gutenberg’s RichText features provides a set of default format options, such as Highlight, Strikethrough and Subscript, etc.
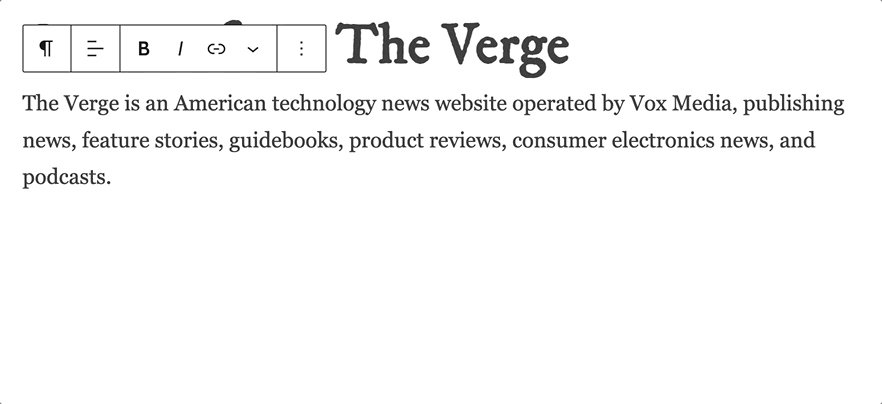
Gutenberg has an API method called registerFormatType
which allows us to register our own format types.
Let us set a goal for the sake of learning.
Goal: Register a new format type called text-shadow that adds a red color text shadow to the selected text.
Registering the custom format
import { registerFormatType } from "@wordpress/rich-text";
const TextShadowFormatter = ( props ) => {
/**
* Contains data about the Richtext content,
* start and end pointers of the text selection.
*/
const value = props.value;
/**
* The callback method to be called when the
* format is applied or removed.
*/
const onChange = props.onChange;
/**
* A boolean that determines if the format is
* applied on the selected text. It's value changes
* when applyFormat, removeFormat or toggleFormat
* is called.
*
* This value is not mandatory, but helpful to
* highlight the ToolBar button that a formatting
* option has been added.
*/
const isActive = props.isActive;
return null;
};
registerFormatType( 'my-namespace/text-shadow', {
title: __( 'Text shadow' ),
tagName: 'span',
className: 'my-namespace-text-shadow',
edit: TextShadowFormatter,
} );
Code language: JavaScript (javascript)
We have registered a format type called text-shadow
under the my-namespace
namespace. When you search for this format type, it will be labeled as Text Shadow in the dropdown.
The CSS text-shadow is applied to the .my-namespace-text-shadow
CSS class. I am not adding the CSS as it is very simple to do so.
The most important bit is the component <TextShadowFormatter />
. This component will hold the logic of applying/removing/toggling the format.
Adding the logic to apply the custom format
import { registerFormatType, toggleFormat } from "@wordpress/rich-text";
import { RichTextToolbarButton } from '@wordpress/block-editor';
const TextShadowFormatter = ( props ) => {
/**
* Contains data about the Richtext content,
* start and end pointers of the text selection.
*/
const value = props.value;
/**
* The callback method to be called when the
* format is applied or removed.
*/
const onChange = props.onChange;
/**
* A boolean that determines if the format is
* applied on the selected text. It's value changes
* when applyFormat, removeFormat or toggleFormat
* is called.
*
* This value is not mandatory, but helpful to
* highlight the ToolBar button that a formatting
* option has been added.
*/
const isActive = props.isActive;
return (
<RichTextToolbarButton
title={ __( "Text shadow" ) }
onClick={ () => {
/**
* toggleFormat accepts 2 arguments:
* RichTextValue: value
* RichTextFormat: format
*/
const toggledFormat = toggleFormat( value, {
type: 'my-namespace/text-shadow',
} );
onChange( toggledFormat );
} }
isActive={ isActive }
/>
);
};
registerFormatType( 'my-namespace/text-shadow', {
title: __( 'Text shadow' ),
tagName: 'span',
className: 'my-namespace-text-shadow',
edit: TextShadowFormatter,
} );
Code language: JavaScript (javascript)
Final result
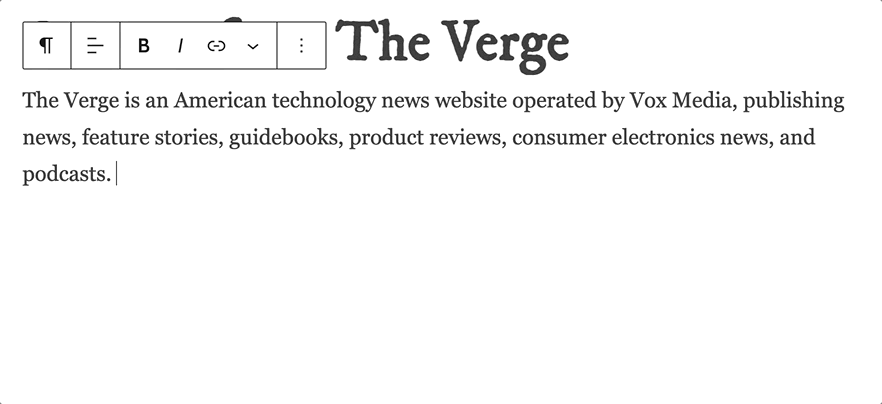