This is what we are going to do through code.
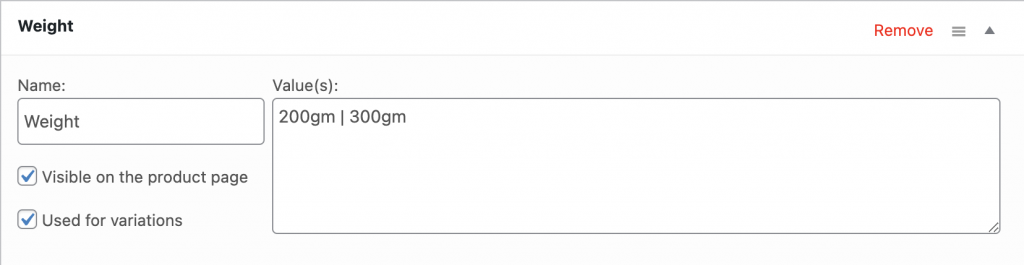
When you add attributes to a product through the product edit screen (above) or the code (below), it only adds the attribute data to the product meta. By design, it does not add the attribute under Products > Attributes.
In our example, we have a product with ID 10
and we are going to add an attribute called “Weight” with the attribute terms 200gm
and 300gm
.
<?php
// Get the product with the ID 10.
$product = wc_get_product( 10 );
// The attribute name.
$attribute_name = 'Weight';
// We start building the attribute starting here.
$attribute = new \WC_Product_Attribute();
// Set the attribute name.
$attribute->set_name( $attribute_name );
// Set the attribute terms.
$attribute->set_options( array(
'200gm',
'300gm',
) );
// Set to `true` so that it can be used for variations.
$attribute->set_variation( true );
// Set to `true` so that it is visible on the product page.
$attribute->set_visible( true );
/* We will only set the new attibute if
* the product doesn't have it already.
*/
// Get all the attributes assigned to our product.
$all_attributes = $product->get_attributes();
// Get the slug equivalent of our attribute name.
$attribute_slug = wc_sanitize_taxonomy_name( $attribute_name );
/* Return if the product already has an attribute
* with the slug `$attribute_slug`.
*/
if ( isset( $all_attributes[ $attribute_slug ] ) ) {
return;
}
// Set the new attribute on the product.
$product->set_attributes(
wp_parse_args(
array(
$attribute
),
$all_attributes
)
);
// Save the product.
$product->save();
Code language: PHP (php)
Hope this helped!