As developers, we love concise code. A single line of elegant syntax feels like a small victory—less typing, more readability, and often, better performance. But is shorter syntax always the fastest? The answer is a surprising no. Let’s dive into a common scenario to understand why.
The Problem: Finding the Length of the Last Word
The definition of a word is simple: a sequence of non-space characters. Our task is to find the length of the last word in a string.
A short, seemingly elegant solution in Python might look like this:
s = "Hello, world"
print(len(s.split()[-1]))
Code language: Python (python)
This method splits the string into words using spaces as delimiters, then takes the last word and measures its length. Clean, right?
What if I told you the faster solution has more lines of code, looks slightly less elegant, but performs significantly better?
The larger, yet faster solution
s = "Hello, world"
s = s.strip()
print(len(s[s.rfind(' ') + 1:]));
Code language: Python (python)
Here, instead of splitting the string, we locate the last space using rfind
, slice the string from that point onward, and calculate the length of the resulting substring.
At first glance, the first solution feels more intuitive and Pythonic. But in a performance showdown, the results might surprise you.
Performance Testing
Let’s compare these two approaches for strings of varying sizes. Here are the results (measured in microseconds):
String Size | split + indexing | rfind + slicing |
---|---|---|
1 | 0.844 | 1.04 |
10 | 1.28 | 1.04 |
100 | 7.18 | 1.08 |
250 | 14.0 | 1.06 |
500 | 32.4 | 1.07 |
For larger strings, the difference becomes dramatic. While split + indexing
slows down significantly as the input grows, rfind + slicing
remains remarkably constant.
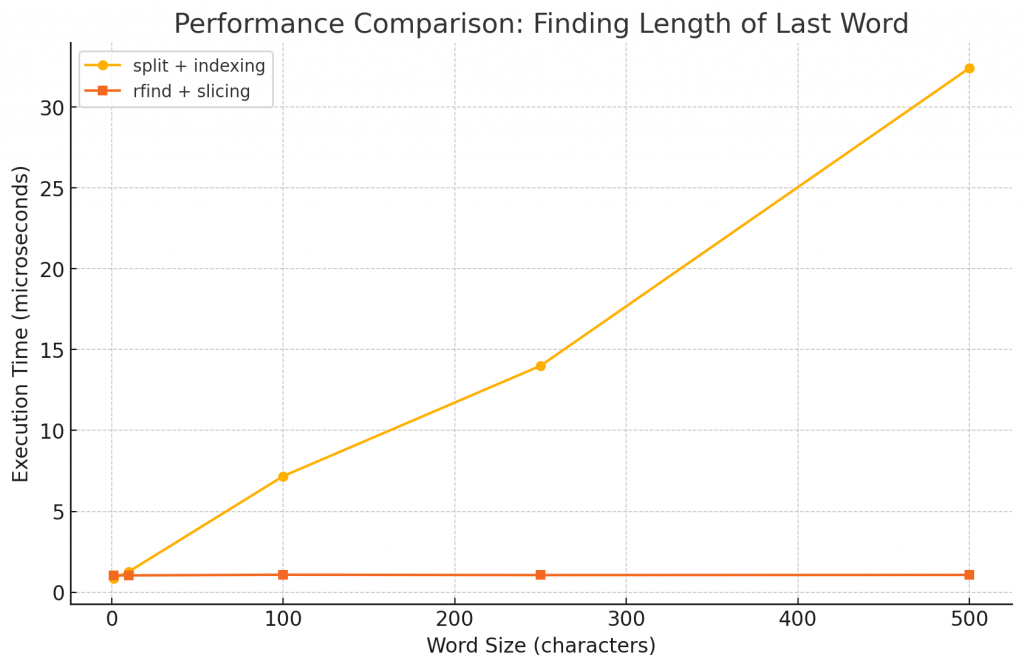
Why the Difference?
The culprit is split()
, which creates an array of all the words in the string. This step adds both time and memory overhead, especially for larger inputs. In contrast, rfind
directly finds the last space in the string without creating new data structures, making it faster and more memory-efficient.
The Lesson: Readability vs. Performance
Short syntax, while appealing, often hides inefficiencies. In the case of split + indexing
, the trade-off for readability is performance—an acceptable cost for small strings but problematic for larger datasets or performance-critical code.
Does this mean you should avoid short syntax? Absolutely not. Readability is a core tenet of good software development. But understanding the trade-offs allows you to make informed decisions. When performance matters, always test and profile your code.
But is this the same with all Languages?
We’ve seen how a longer, more explicit solution in Python can outperform a shorter, more intuitive one when it comes to performance. But does this behavior hold true across other programming languages?
Interestingly, the answer is no — not always.
Let’s take JavaScript as an example. Here are two equivalent solutions for finding the length of the last word:
// Solution #1
console.log(string.split(' ').at(-1).length);
// Solution #2
console.log(string.length - string.lastIndexOf(' ') - 1);
Code language: JavaScript (javascript)
At first glance, you might expect Solution 2 to be faster since it avoids creating an array, just like in Python. However, performance testing reveals that both solutions perform almost the same in JavaScript. This is because JavaScript has highly optimized techniques for string-to-array conversions, making operations like split()
exceptionally fast even for larger inputs.
Conclusion
Short syntax is a powerful tool, but it’s not a silver bullet. As developers, our goal is to write code that is not only functional and readable but also performant. Sometimes, this means embracing solutions that are less concise but more efficient.
However, writing performant code isn’t just about picking the longer or shorter syntax — it’s about understanding how the language works under the hood. By learning the design choices and internal optimizations of the programming languages we use, we can make informed decisions rather than relying solely on intuition or readability.
The next time you’re tempted by a one-liner, remember: shortcuts might save you typing, but they don’t always save time.
Leave a Reply